Phew, the last few articles have been pretty technical, so lets have a nice gameplay-focused one today.
We'll go over the creatures, how they're designed, developed and implemented. We'll also talk about moves and their effects, and we might also touch on the items further down.
Premise
Egg Trainer allows you to buy and hatch eggs, train the creatures inside, and battle them against real life opponents. Each creature has a set number of moves, as well as species-specific statistics (try saying that three times fast). On top of that, genetics and training play into a specific creature's final stats.
If this sounds like Pokemon, you're right on the money - it was heavily inspired by it.
Basics
The basic creature concept begins with an idea - this can be something as simple as a pun, or for a lot of the common creatures, can be something like a fusion of two animals. We have an internal document called "thumbnails.png" which actually lists a number of ideas, simply by outlining their silhouettes.
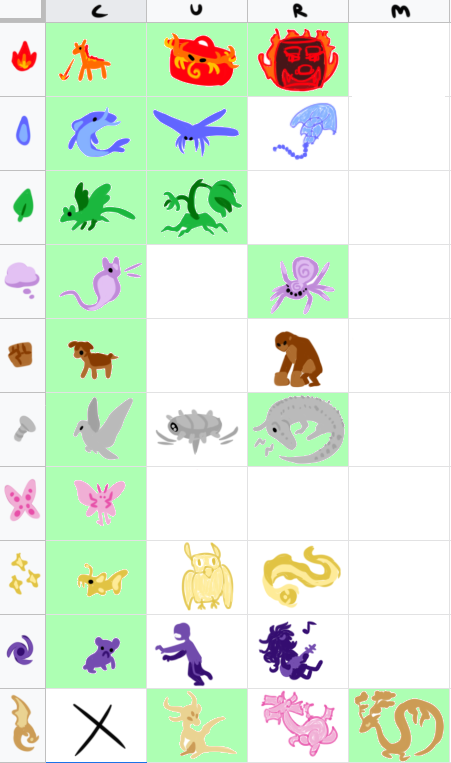
(I've removed some mythic concepts because I want to keep the mythics under wraps.)
They're divided along their types and rarities - common, uncommon, rare and mythic. There's no evolution in this game, so what you see is what you get. Not all of the creatures have been planned, or even illustrated yet - we're still deep in development.
Each week, my artist or I chooses a concept on the thumbnails document to begin working on. We brainstorm a name for the creature, some backstory/lore, and my artist begins illustrating that creature. They share the line art throughout to ensure I'll like the final result, and finally they usually deliver at the end of the week, at which point we discuss more ideas or concepts, or even alter existing ones (you'll notice that the rare dragon is pink, for example - that one was originally drawn in the fairy row, for instance).
Some ideas do get outdated or rejected entirely (like the protog, which was replaced by the puggy), but generally we're not far enough through development to throw too much away.
Elements
First, lets discuss the creatures' elemental types. There are 10 elements in Egg Trainer, ranging from the classic fire-water-grass triangle, to more unusual types like fae and dragon. Each type has a number of strengths and weaknesses. Below is a JSON array defining those strengths and weaknesses (outer is attacker, inner is defender):
{
"fire": {
"water": 0.5, "grass": 2, "dark": 2
},
"water": {
"fire": 2, "grass": 0.5, "light": 2
},
"grass": {
"fire": 0.5, "water": 2, "fae": 2
},
"psychic": {
"fighting": 2, "metal": 0.5
},
"fighting": {
"psychic": 2, "fae": 0.5
},
"metal": {
"fae": 2, "water": 0.5
},
"fae": {
"dark": 2, "light": 0.5
},
"light": {
"dark": 2, "dragon": 0.5
},
"dark": {
"dragon": 2, "fire": 0.5
},
"dragon": {
"fire": 2, "psychic": 2, "fae": 0.5, "dark": 0.5
}
}
The strengths and weaknesses are strictly 2x and 0.5x at the moment, but these can in theory be tweaked later during development or even post launch. I'd like the exact balancing of elemental types, creatures, etc. to be publicly available knowledge, so that no one person has an inside advantage against another (maybe a fan-driven wiki would be good?).
Moves
Next, let's look at moves. There's about 20 moves so far, so I won't post them all - let's look at a few examples instead.
{
"tackle": {
"name": "Tackle",
"description": "The user charges into the target.",
"type": "physical",
"element": "fighting",
"damage": 5,
"value": 10
},
"firespit": {
"name": "Fire Spit",
"description": "The user spits fire at their opponent.",
"type": "physical",
"element": "fire",
"damage": 15,
"value": 10,
"meta": {
"signature": "spitty"
}
},
"meteor": {
"name": "Meteor",
"description": "The user summons a fireball from the sky.",
"type": "magical",
"element": "fire",
"damage": 10,
"value": 10
},
...
}
It's another JSON structure! The keys are unchanging references to specific moves - these are what are used in the creature structures. They have a name (which can be different from the key), a description, a type (either physical or magical), and an element (one of the 10 listed above). Magical and Physical will eventually have an impact, based on other contextual elements of the battle. Physical typed moves use the creature's strength, while magical typed attacks use the creature's power - and they may have other contextual effects. I'd like certain elements to lean towards one over the other, but it's not essential.
Finally, they each have a base damage value and cost value (here called "value" - that needs fixing). These need to be balanced later in development, during beta testing.
There's also the "meta" structure, which contains contextual information, which may be absent. For example, if a move is a signature of a specific creature (or specific type), then it is listed here ("fire spit" is the signature of "spitty"). Tools can be used later to double check consistency.
The meta structure will also contain any serialized effect functions that moves may have (such as boosting stats in certain whether, etc). This can be done by saving the JavaScript code as text, and deserializing it - strange, but it works.
Species
The "species" of a creature is a large determining factor of what that creature can do, and even what it is. The species structure also contains the egg data about that species in particular.
{
"spitty": {
"name": "Spitty",
"element": "fire",
"description": "A llama-like creature with fire-infused wool. It spits fire at anything that annoys it.",
"egg": {
"rarity": "common",
"value": 200
},
"stats": {
"health": 10,
"speed": 10,
"physicalAttack": 10,
"physicalDefense": 10,
"magicalAttack": 10,
"magicalDefense": 10
},
"moves": [ "tackle", "firespit", "babyeyes" ],
"frontImage": "spitty.png"
},
"whalephant": {
"name": "Whalephant",
"element": "water",
"description": "A majestic deep-sea creature, known for never forgetting. It has a prehensile nose.",
"egg": {
"rarity": "common",
"value": 200
},
"stats": {
"health": 10,
"speed": 10,
"physicalAttack": 10,
"physicalDefense": 10,
"magicalAttack": 10,
"magicalDefense": 10
},
"moves": [ "splash", "watercannon", "babyeyes", "babyfat", "thunderclap" ],
"frontImage": "whalephant.png"
},
...
}
The structure (which currently only contains the 9 common creatures, but we're cutting it down anyway) also use JSON. It's JSON all the way down!
Each creature has a display name, it's element (creatures only ever have one element), a description, the egg data (such as it's rarity and that egg's cost... which probably should be moved to an external structure), the stats block, a list of moves stored in an array, and the image file name (named frontImage, because I originally thought there'd be more than one).
The stats block contain the base stats for that specific species - it's health, speed, physical attack, physical defense, magical attack and magical defense. The moves are just a list of keys, referencing the keys from the previous section.
Again, these all have dummy stats, which will be balanced later in the game's development, when combat is working.
Creatures
Again, I've fallen into technical talk, but at least you know what goes into creating a specific species of creature. In general, I want to design a well-rounded roster, with rarer creatures having slightly stronger stats, but with openings for counter play from lower rarities.
So lets look at the structure for individual creatures (pseudocode this time, because the real thing is ugly SQL):
creature:
profileId
nickname
species
geneticPoints:
health
speed
strength
power
trainingPoints:
health
speed
strength
power
trainingFinish
trainingType
breeding
The profileId simply references which profile owns the creature. The Nickname is a field for storing a custom name, but was never implemented (I'm not sure why). Then you have the species of the creature, indicating a lot about it (such as some of it's stats, and it's moves, etc.)
Next, you have it's genetics - these don't change throughout a creature's life, but they do largely carry down from parents to children. The process as thus:
- Take 1 random stat from the mother
- Take 1 random stat from the father
- Take 1 random stat from either parent
- Randomize the last stat
Which creature is the mother and father is largely unimportant (the creatures don't have genders). The genetic stats can be between 0 and, I think 15? But that may change.
The next part is training - each creature has a set number of points (16, to be exact) that they can allocate between their stats to customize them (each time you allocate to a specific stat, it takes a number of minutes equal to that stat's new value).
"trainingFinish" is the finish time of the training, and is null if the creature isn't training. The "trainingType" is which stat is being trained (health, speed, power or strength). Finally, breeding is if this creature is breeding or not.
The creature's purchased moves are stored in their own database table.
Eggs
Players buy eggs from the in-game shop using in-game coins. They then hatch these eggs over a period of time, using an incubator. Players have one incubator to begin with, and can buy more as they progress. This is a basic limit on the number of creatures that can enter the ecosystem at a time, based on the number of players.
Each egg has a specific element and rarity, which can be determined simply by looking at it. However what kind of creature hatches from that egg is randomly chosen from that element and rarity, so you can theoretically have more than one creature in a specific thumbnails.png slot (this will come way later in development).
Apart from this, the eggs also hold genetic data which is passed onto the hatched creature.
Items
There are two types of items - normal items and premium items. Normal items can be purchased using in-game coins, while premium items require microtransactions to obtain. Their data is stored separately, but generally they're identical in structure.
{
"potion": {
"name": "Potion",
"type": "consumable",
"value": 50,
"sprite": "potion.png",
"description": "For healing creatures during battles. Single use.",
"shop": "constant",
"meta": {
"effect": "heal",
"value": 10
}
},
...
}
Here we see a potion object, with an immutable key that can be referenced elsewhere, a name, the type (types are "static" and "consumable"), the value (in in-game coins), the sprite file name, the description (which honestly, should be placed under the name), the "shop" value (which, while unimplemented, will be either "constant" or "rotate"), and a contextual meta structure.
The meta field contains things like the effect of the item ("heal", "hatch", etc.) and any other values needed by that item. There is also a "minQuanity" and "maxQuantity" field in the item's body that needs to be moved to the meta structure (you must have between 1 and 2 incubators, currently).
{
"goldenapple": {
"name": "Golden Apple",
"type": "consumable",
"value": 199,
"sprite": "goldenapple.png",
"description": "For healing creatures during battles. Single use.",
"shop": "constant",
"premium": true,
"meta": {
"effect": "heal",
"value": 10
}
},
...
}
Here, you can see a premium item - this is what you pay for with real money. The fields are largely the same; in fact, golden apples are duplicates of potions. The only difference is the "premium" field, which is set to "true", and the "value" field, which list the price in cents, AUD. (I'm currently using Australian dollars because I was inexperienced with PayPal's API first time round - I'm hoping to change it to USD.)
Premium items are theoretically how I'll support the game's development costs. Consumable items will be single-use for each combat - you can only use one potion for the entire battle. This is what makes golden apples desirable - they're essentially an extra potion. My intent is not to create a pay-to-win situation, but rather a risk-vs-reward situation: should you spend your entire move to use a premium item, and potentially risk other elements of the battle?
Conclusion
Wow, another massive article over. Hopefully you understand what goes into making a specific species of creature, as well as what makes up the creatures in general. Also, the items were a bonus throw-in. This did become technical with JSON structures again, but hopefully JSON is understandable by most readers (and I hopefully explained each one well enough).
My name is Kayne Ruse of KR Game Studios, you can find me on the net as Ratstail91, usually researching something. Have you ever heard of the MDA framework?
Egg Trainer Website: https://eggtrainer.com/
MERN-template repo: https://github.com/krgamestudios/MERN-template
news-server repo: https://github.com/krgamestudios/news-server
Business Inquiries: [email protected]